[iOS] Interview Experience at Expedia
[iOS] Interview Experience at Expedia
1. Letter Combinations of a Phone Number
Given a string containing digits from
2-9
inclusive,
return all possible letter combinations that the number could
represent.
A mapping of digit to letters (just like on the telephone buttons) is given below. Note that 1 does not map to any letters.
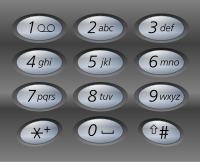
Example:
Input: "23"
Output: ["ad", "ae", "af", "bd", "be", "bf", "cd", "ce", "cf"].
Note: Although the above answer is in lexicographical order, your answer could be in any order you want.
Practise: https://leetcode.com/problems/letter-combinations-of-a-phone-number/
2. Unique Paths II
A robot is located at the top-left corner of a m x n grid (marked ‘Start’ in the diagram below).
The robot can only move either down or right at any point in time. The robot is trying to reach the bottom-right corner of the grid (marked ‘Finish’ in the diagram below).
Now consider if some obstacles are added to the grids. How many unique paths would there be?

An obstacle and empty space is marked as
1
and
0
respectively
in the grid.
Note: m and n will be at most 100.
Example 1:
Input:
[
[0,0,0],
[0,1,0],
[0,0,0]
]
Output: 2
Explanation:
There is one obstacle in the middle of the 3x3 grid above.
There are two ways to reach the bottom-right corner:
1. Right -> Right -> Down -> Down
2. Down -> Down -> Right -> Right
Practise: https://leetcode.com/problems/unique-paths-ii/
3. Sliding Window Maximum
Given an array nums, there is a sliding window of size k which is moving from the very left of the array to the very right. You can only see the k numbers in the window. Each time the sliding window moves right by one position. Return the max sliding window.
Follow up:
Could you solve it in linear time?
Example:
Input: nums =[1,3,-1,-3,5,3,6,7]
, and k = 3
Output:[3,3,5,5,6,7]
Explanation:
Window position Max
--------------- -----
[1 3 -1] -3 5 3 6 7 3
1 [3 -1 -3] 5 3 6 7 3
1 3 [-1 -3 5] 3 6 7 5
1 3 -1 [-3 5 3] 6 7 5
1 3 -1 -3 [5 3 6] 7 6
1 3 -1 -3 5 [3 6 7] 7
Constraints:
-
1 <= nums.length <= 10^5
-
-10^4 <= nums[i] <= 10^4
-
1 <= k <= nums.length
Practise: https://leetcode.com/problems/sliding-window-maximum/
Hope you like this article & is useful for people looking to solve question asked in iOS interview at various organisation, Please ❤️ to recommend this post to others 😊. Let me know your feedback. :)