Top IOS Interview Questions and Answers (May 2019) Part I
Top IOS Interview Questions and Answers (May 2019) Part I
Next will be coming soon!!!
Q1. What is dynamic ?
You can use the
@synthesize
and
@dynamic
directives in
@implementation
blocks to trigger specific compiler actions.
Important:
If you do not specify either
@synthesize
or
@dynamic
for a
particular property, you must provide a getter and setter (or
just a getter in the case of a
readonly
property) method implementation for that property. If you do
not, the compiler generates a warning.
@dynamic
You use the
@dynamic
keyword to tell the compiler that you will fulfill the API
contract implied by a property either by providing method
implementations directly or at runtime using other mechanisms
such as dynamic loading of code or dynamic method resolution. It
suppresses the warnings that the compiler would otherwise
generate if it can’t find suitable implementations. You should
use it only if you know that the methods will be available at
runtime.
The example shown in Listing 1.0 illustrates using
@dynamic
with a
subclass of
NSManagedObject
.
Listing 1.0 Using @dynamic with NSManagedObject
@interface MyClass : NSManagedObject
@property(nonatomic, retain) NSString *value;
@end
@implementation MyClass
@dynamic value;
@end
NSManagedObject
is provided by the Core Data framework. A managed object class
has a corresponding schema that defines attributes and
relationships for the class; at runtime, the Core Data framework
generates accessor methods for these as necessary. You therefore
typically declare properties for the attributes and
relationships, but you don’t have to implement the accessor
methods yourself and shouldn’t ask the compiler to do so. If you
just declared the property without providing any implementation,
however, the compiler would generate a warning. Using
@dynamic
suppresses the warning.
Q2. ViewDidLoad vs ViewDidAppear (How to use them appropriately).
ViewDidLoad: This method is called after the view controller has loaded
its view hierarchy into memory. This method is called regardless
of whether the view hierarchy was loaded from a nib file or
created programmatically in the
loadView()
method. You usually override this method to perform additional
initialization on views that were loaded from nib files.
ViewDidAppear: Notifies the view controller that its view was added to a
view hierarchy. You can override this method to perform
additional tasks associated with presenting the view. If you
override this method, you must call
super
at some
point in your implementation.
Note: If a view controller is presented by a view controller inside of a popover, this method is not invoked on the presenting view controller after the presented controller is dismissed.
Q3.What is UIResponder chain?
Apps receive and handle events using
responder objects. A
responder object is any instance of the
UIResponder
class, and common subclasses include
UIView
,
UIViewController
, and
UIApplication
. Responders receive the raw event data and must either handle
the event or forward it to another responder object. When your
app receives an event, UIKit automatically directs that event to
the most appropriate responder object, known as the
first responder.
Figure 1: Responder chains in an app

If the text field does not handle an event, UIKit sends the
event to the text field’s parent
UIView
object,
followed by the root view of the window. From the root view, the
responder chain diverts to the owning view controller before
directing the event to the window. If the window cannot handle
the event, UIKit delivers the event to the
UIApplication
object, and possibly to the app delegate if that object is an
instance of
UIResponder
and
not already part of the responder chain.
Q4.GCD vs NSOperation ?
Grand Central Dispatch, a concurrency framework, first deployed by Apple in OS X 10.6
and iOS 4.
• Queues and Tasks
• Supporting Concept
• Blocks, an extension to C, used heavily by Grand Central
Dispatch
NSOperation, a higher-level API that allows OS X and iOS applications to
perform tasks concurrently
• This higher-level API existed
before Grand Central Dispatch
• but has since been
re-implemented to use GCD underneath.
There are three approaches to the design of concurrent systems
•
Shared Mutability
: Multiple threads can access mutable variables; need
synchronization
•
Isolated Mutability
: Multiple threads; but only one thread can access a mutable
variable at a time; no locking; the Actor Model (in other
languages besides Elixir)
•
Pure Immutability
: No mutable variables; values can be shared freely across
multiple threads
The
first key abstraction in GCD
is the task
• A task is a discrete goal that your
application wants to accomplish like display a web page, analyze
a set of tweets, calculate primes
• Tasks can depend on
other tasks and might be decomposed into sub-tasks
• Tasks
themselves are ultimately decomposed into work items. work items
are chunks of code (known as blocks) that need to be executed to
make progress on a task like read a file, parse a tweet, break
down a range of numbers
• Work items get placed on queues;
when they get to the front of a queue, they are assigned to a
thread and get executed
The second key
abstraction for GCD
is the queue
- Queues can be either a
serial queue, created for a specific application or a
global queue, created by the operating system
- Three global queues
of different
priorities: low, default, and high
- Serial queues are lightweight
constructs; you can have as many as you need. Each serial queue
must “target” a global queue to get work done. Serial queues can
also target other serial queues but ultimately they have to
point at a global queue.
Both types of queues have FIFO semantics, difference lies in the semantics of work completion :
a. Global queues : dispatch (assign work items to threads) in FIFO order
b. Serial queues : dispatch and complete work items in FIFO order
What does this mean?
- A serial queue executes only one work item at a time
- A global queue executes many work items at a time
Q5.Can we use multiple UIWindow in a single app?
Most apps need only one window, which displays the app’s content on the device’s main screen. Although you can create additional windows on the device’s main screen, extra windows are commonly used to display content on an external screen, as described in Displaying Content on a Connected Screen.
Q6.What is category ?
You use categories to define additional methods of an existing class — even one whose source code is unavailable to you — without subclassing. You typically use a category to add methods to an existing class, such as one defined in the Cocoa frameworks. The added methods are inherited by subclasses and are indistinguishable at runtime from the original methods of the class. You can also use categories of your own classes to:
- Distribute the implementation of your own classes into separate source files — for example, you could group the methods of a large class into several categories and put each category in a different file.
- Declare private methods.
You add methods to a class by declaring them in an interface file under a category name and defining them in an implementation file under the same name. The category name indicates that the methods are an extension to a class declared elsewhere, not a new class.
Q7. Delegate and protocol
Delegation is a simple and powerful pattern in which one object in a program acts on behalf of, or in coordination with, another object. The delegating object keeps a reference to the other object — the delegate — and at the appropriate time sends a message to it. The message informs the delegate of an event that the delegating object is about to handle or has just handled. The delegate may respond to the message by updating the appearance or state of itself or other objects in the application, and in some cases it can return a value that affects how an impending event is handled. The main value of delegation is that it allows you to easily customize the behavior of several objects in one central object.
Data Source
A data source is almost identical to a delegate. The difference is in the relationship with the delegating object. Instead of being delegated control of the user interface, a data source is delegated control of data.
Protocol
A protocol declares a programmatic interface that any class may choose to implement. Protocols make it possible for two classes distantly related by inheritance to communicate with each other to accomplish a certain goal. They thus offer an alternative to subclassing.
Formal and Informal Protocols
There are two varieties of protocol, formal and informal:
-
A formal protocol declares a list of methods that client
classes are expected to implement. Formal protocols have their
own declaration, adoption, and type-checking syntax. You can
designate methods whose implementation is required or optional
with the
@required
and@optional
keywords. Subclasses inherit formal protocols adopted by their ancestors. A formal protocol can also adopt other protocols. Formal protocols are an extension to the Objective-C language. -
An informal protocol is a category on
NSObject
, which implicitly makes almost all objects adopters of the protocol. (A category is a language feature that enables you to add methods to a class without subclassing it.) Implementation of the methods in an informal protocol is optional. Before invoking a method, the calling object checks to see whether the target object implements it. Until optional protocol methods were introduced in Objective-C 2.0, informal protocols were essential to the way Foundation and AppKit classes implemented delegation.
Q8. Application life cycle
Most state transitions are accompanied by a corresponding call to the methods of your app delegate object. These methods are your chance to respond to state changes in an appropriate way. These methods are listed below, along with a summary of how you might use them.
-
application:willFinishLaunchingWithOptions:
—This method is your app’s first chance to execute code at launch time. -
application:didFinishLaunchingWithOptions:
—This method allows you to perform any final initialization before your app is displayed to the user. -
applicationDidBecomeActive:
—Lets your app know that it is about to become the foreground app. Use this method for any last minute preparation. -
applicationWillResignActive:
—Lets you know that your app is transitioning away from being the foreground app. Use this method to put your app into a quiescent state. -
applicationDidEnterBackground:
—Lets you know that your app is now running in the background and may be suspended at any time. -
applicationWillEnterForeground:
—Lets you know that your app is moving out of the background and back into the foreground, but that it is not yet active. -
applicationWillTerminate:
—Lets you know that your app is being terminated. This method is not called if your app is suspended.
Q9. Categories limitations ?
You cannot safely override methods already defined by the class itself or another category.
Q10.What is the blocks ?
Block objects are a C-level syntactic and runtime feature.
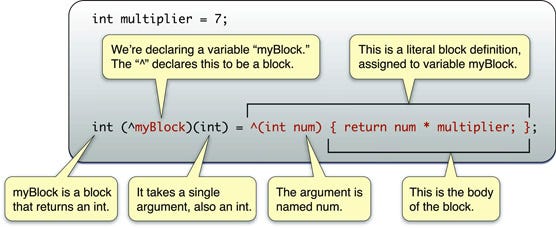
Bonus:
VIPER offers a few key advantages to MVC. First, it offers more abstraction. The Presenter contains presentation logic that glues business logic with view logic. The Interactor handles purely data manipulation and verification. This includes making service calls to the backend to manipulate state, such as sign in and request a trip. And finally, the Router initiates transitions, such as taking the user from home to confirmation screen. Secondly, with the VIPER approach, the Presenter and Interactor are plain old objects, so we can use simple unit tests.