Top IOS Interview Questions and Answers (April 2019) Part IV
Blocks
A block may capture values from local variables; when this
occurs, memory must be dynamically allocated. The initial
allocation is done on the stack, but the runtime provides a
Block_copy
function which, given a block pointer, either copies the
underlying block object to the heap, setting its reference count
to 1 and returning the new block pointer, or (if the block
object is already on the heap) increases its reference count by
1. The paired function isBlock_release
, which decreases the reference count by 1 and destroys the
object if the count reaches zero and is on the heap.
Property declarations
-
assign
implies__unsafe_unretained
ownership. -
copy
implies__strong
ownership, as well as the usual behavior of copy semantics on the setter. -
retain
implies__strong
ownership. -
strong
implies__strong
ownership. -
unsafe_unretained
implies__unsafe_unretained
ownership. -
weak
implies__weak
ownership.
@autoreleasepool
To simplify the use of autorelease pools, and to bring them
under the control of the compiler, a new kind of statement is
available in Objective-C. It is written
@autoreleasepool
followed by a
compound-statement,
i.e. by a new scope delimited by curly braces. Upon entry to
this block, the current state of the autorelease pool is
captured. When the block is exited normally, whether by
fallthrough or directed control flow (such as
return
or
break
), the
autorelease pool is restored to the saved state, releasing all
the objects in it. When the block is exited with an exception,
the pool is not drained.
@autoreleasepool
may be used in non-ARC translation units, with equivalent
semantics.
Method Swizzling
Sometimes for convenience, sometimes to work around a bug in a framework, or sometimes because there’s just no other way, you need to modify the behavior of an existing class’s methods. Method swizzling lets you swap the implementations of two methods, essentially overriding an existing method with your own while keeping the original around.
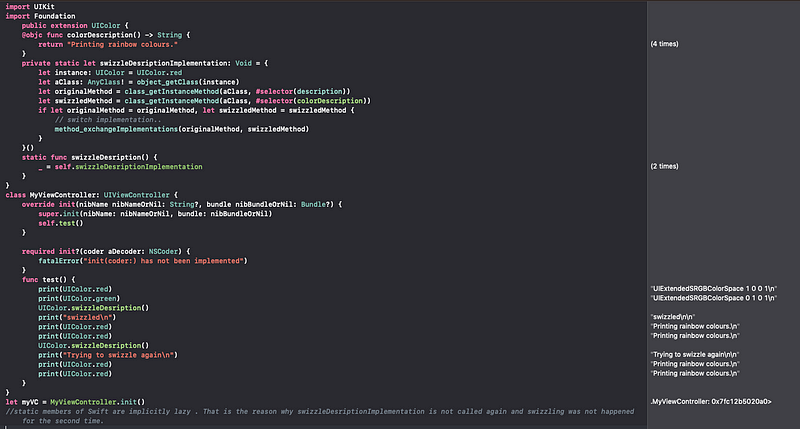
Objective-C vs Swift comparison
Objective-C uses the runtime code compilation
Objective-C isn’t a fast language. The main reason is that it uses the runtime code compilation, rather than the compile time. This means that when the Objective-C object calls for another object in the code, there is an extra level of indirection involved. Generally, this happens very fast but when the code compilation happens a significant number of times, it becomes measurable. Objective-C is a superset of C and all C functions that you will write in Objective-C will be just as fast. Developers who write the performance-sensitive code often go back to clear C for those inner loops.
Safety
Swift was designed to improve the code safety for iOS products. It was created as a type-safe and memory-safe language. Type safety means that the language itself prevents type errors. The importance of type memory safety is that it helps avoid vulnerabilities associated with dangling or uninitialized pointers. These types of errors are the most common in development and difficult to find and debug. These advantages of the Swift language make it more attractive.
Maintenance
Managing files in Objective-C is a frustrating process because developers must manage two separate files. While Swift requires less maintenance and doesn’t require you to manage two files. The thing is that Swift automatically completes the reliances and performs an incremental build in the file.
Memory management
Objective-C language supports the Automatic Reference Counting (ARC) inside of the object-oriented code itself. The issue is that it cannot access C code and other APIs as Core Graphics. On the contrary, Swift is more consolidated, and its ARC is complete for procedural and object-oriented paths. Due to this fact, huge leaks of memory with the Swift language are impossible.
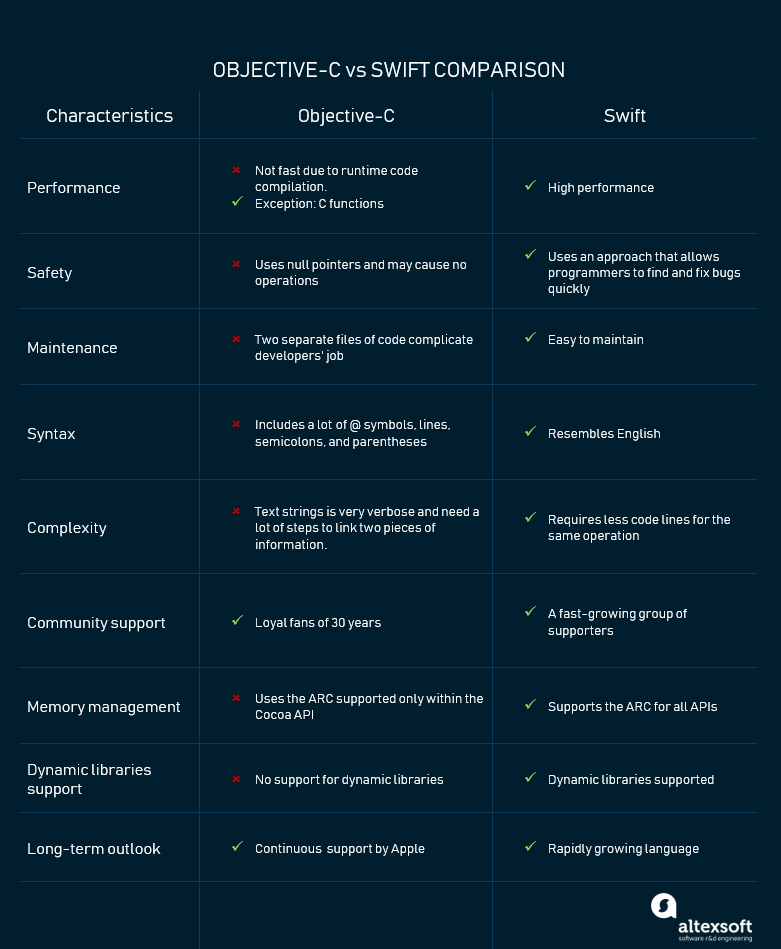
Difference between block (Objective C) and closure (Swift)
Swift closures and Objective-C blocks are compatible, so you can pass Swift closures to Objective-C methods that expect blocks. Swift closures and functions have the same type, so you can even pass the name of a Swift function.
Closures have similar capture semantics as blocks but differ in one key way: Variables are mutable rather than copied. In other words, the behavior of __block in Objective-C is the default behavior for variables in Swift.
Associated Types
An
associated type gives a
placeholder name to a type that is used as part of the protocol.
The actual type to use for that associated type isn’t specified
until the protocol is adopted. Associated types are specified
with the
associatedtype
keyword.
protocol Container {
associatedtype
Item
mutating func append(_ item: Item)
var count: Int
{ get }
subscript(i: Int) -> Item { get }
}

The definition of
typealias Item = Int
turns the abstract type of
Item
into a
concrete type of
Int
for this
implementation of the
Container
protocol.
Thanks to Swift’s type inference, you don’t actually need to
declare a concrete
Item
of
Int
as part of
the definition of
conformingClass.
References: