Top IOS Interview Questions and Answers (April 2019) Part I
-
What is Cocoa?
Cocoa and Cocoa Touch are the application development environments for OS X and iOS, respectively. Both Cocoa and Cocoa Touch include the Objective-C runtime and two core frameworks:
- Cocoa, which includes the Foundation and AppKit frameworks, is used for developing applications that run on OS X.
- Cocoa Touch, which includes Foundation and UIKit frameworks, is used for developing applications that run on iOS.
3. Difference between Nil & NULL?
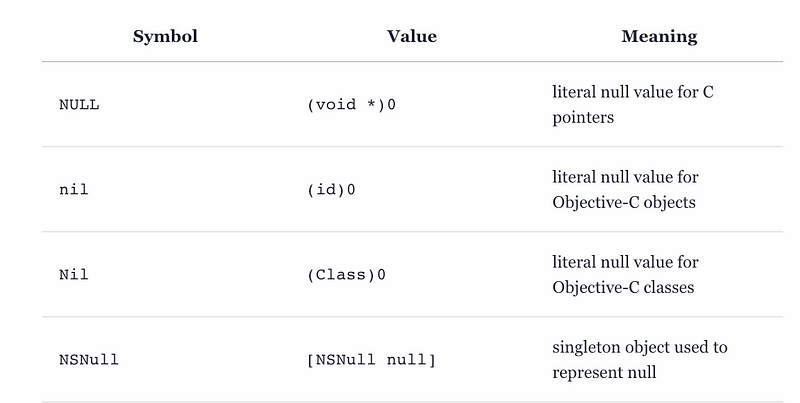
4. Difference between AnyObject & Any?
Any
can
represent an instance of any type at all, including function
types.
Meaning of
AnyObject
and
Any
have
changed with the release of Swift 3.
In Swift 3, the
id
type in
Objective-C now maps to the
Any
type in
Swift, which describes a value of any type, whether a class,
enum, struct, or any other Swift type.
This change improves the compatibility of Swift and Objective-C.
Objective-C collections, for example, can hold elements of
Any
type as of
Swift 3. This means that Foundation's
NSArray
type
can store
String
,
Float
, and
Int
instances.
AnyObject
is a
protocol to which all classes implicitly conform.
You use
AnyObject
when
you need the flexibility of an untyped object or when you use
bridged Objective-C methods and properties that return an
untyped result.
AnyObject
can
be used as the concrete type for an instance of any class, class
type, or class-only protocol.
The
AnyObject
protocol is also useful to bridge the gap between Swift and
Objective-C. Some Objective-C APIs use the
AnyObject
protocol to provide compatibility with Swift.
5. What is @synthesize & @dynamic?
@synthesize will generate getter and setter methods for your property. @dynamic just tells the compiler that the getter and setter methods are implemented not by the class itself but somewhere else (like the superclass or will be provided at runtime).
Mentioning @dynamic with a propertyName tells the compiler not to create accessor methods because user will provide the implementation dynamically in future. Apple allows its developers to provide method implementation at runtime using Dynamic Method Resolution.
6. What is inout parameter?
All parameters passed into a Swift function are
constants, so you can’t
change them. If you want, you can pass in one or more parameters
as inout
, which
means they can be changed inside your function, and those
changes reflect in the original value outside the function.
func doubleInPlace(number: inout Int) {
7. Difference between weak self vs unowned self?
The difference between
unowned
and
weak
is that
weak
is
declared as an Optional while
unowned
is not.
By declaring it
weak
you get to
handle the case that it might be nil inside the closure at some
point. If you try to access an
unowned
variable that happens to be nil, it will crash the whole
program. So only use
unowned
when
you are positive that variable will always be around while the
closure is around.
The only time where you really want to
use
[unowned self]
or
[weak self]
is
when you would create a
strong reference cycle. A strong reference cycle is when there is a loop of ownership
where objects end up owning each other (maybe through a third
party) and therefore they will never be deallocated because they
are both ensuring that each other stick around.
8. What is tuple?
Tuples in Swift occupy the space between dictionaries and structures: they hold very specific types of data (like a struct) but can be created on the fly (like dictionaries). They are commonly used to return multiple values from a function call.
You can create a basic tuple like this:
let person = (name: "Paul", age: 35)
9. When to use delegate or closures?
We can say that delegate callbacks are more process oriented and closure are more results oriented. If you need to be informed along the way of a multi-step process, you’ll probably want to use delegation. If you just want the information you are requesting (or details about a failure to get the information), you should use a closure.
a. If an object has more than one distinct event, use
delegation.
b. If an object is a singleton, we can’t use
delegation.
c. If the object is calling back for additional
information, we’ll probably use delegation.
10. Can we declare variables in protocol and does Protocol support extension?
Yes, The protocol doesn’t specify whether the property should be a stored property or a computed property — it only specifies the required property name and type.

Yes, You can extend an existing type to adopt and conform to a new protocol, even if you don’t have access to the source code for the existing type. Extensions can add new properties, methods, and subscripts to an existing type, and are therefore able to add any requirements that a protocol may demand.
Hope this article is useful for people looking out for a change, This article questions were asked in Athena health and VMWare (5yrs exp iOS engineer), Please ❤️ to recommend this post to others 😊. Let me know your feedback. :)
References:
- https://developer.apple.com/library/archive/documentation/General/Conceptual/DevPedia-CocoaCore/Cocoa.html
- https://nshipster.com/nil/
- https://cocoacasts.com/what-is-any-in-swift
- https://stackoverflow.com/questions/24320347/shall-we-always-use-unowned-self-inside-closure-in-swift
- https://www.hackingwithswift.com/example-code/language/what-is-a-tuple
- https://stablekernel.com/use-blocks-closures-delegates-callbacks/
- https://docs.swift.org/swift-book/LanguageGuide/Protocols.html