The Decorator, Mediator and Bridge Patterns
The Decorator, Mediator and Bridge Patterns
This week we will learn three new patterns, the Decorator, the Mediator and the Bridge.
Decorator Design Pattern
Attach additional responsibilities to an object dynamically. Decorators provide a flexible alternative to subclassing for extending functionality.
- Adapter provides a different interface to its subject. Proxy provides the same interface. Decorator provides an enhanced interface.
In Swift there are two very common implementations of this pattern: Extensions and Delegation.
Cocoa uses the Decorator pattern in the implementation of
several of its classes, including
NSAttributedString
,
NSScrollView
, and
UIDatePicker
.
Mediator Design Pattern
Controller in Model-View-Controller
The Model-View-Controller design pattern assigns roles to the objects in an object-oriented system such as an application. They can be model objects, which contain the data of the application and manipulate that data; they can be view objects, which present the data and respond to user actions; or they can be controller objects, which mediate between the model and view objects. Controller objects fit the Mediator pattern.
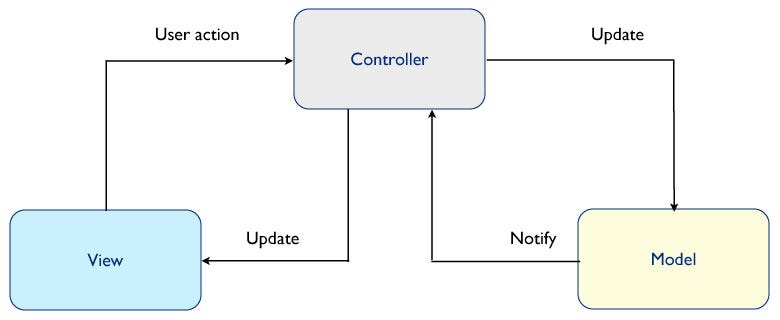
View Controller Transitions
In the “before” diagram above, if ViewControllerA wants to pass myObjectB to ViewControllerC or ViewControllerD, it has to do so by first passing it to ViewControllerB which will later pass it on to ViewControllerC etc. This architecture is prone to producing errors and is a maintenance nightmare.
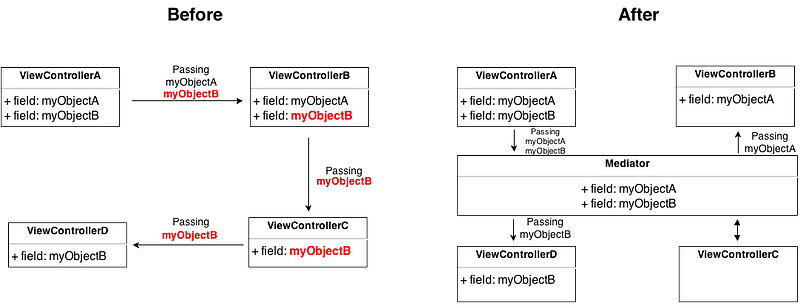
By introducing a Mediator to handle the injections, our problem is solved because the mediator handles the passing of data between any two view controllers now. View controllers C and D no longer depend on ViewControllerB to gain access to a property/object of ViewControllerA. If ViewControllerB has to be replaced, view controllers C and D won’t “break” (unlike before).
Bridge Design Pattern
The bridge pattern is used to separate the abstract elements of a class from the implementation details, providing the means to replace the implementation details without modifying the abstraction.
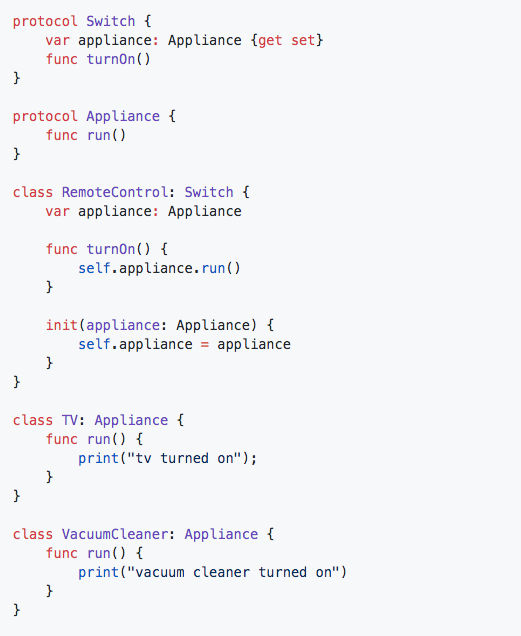
Hope you like it, Please feel free to comment or clap to encourage :)
References: