The Singleton, Facade and Command Patterns
The Singleton, Facade and Command Patterns
Facade Design Pattern, Singleton Design Pattern, Command Design Pattern, Swift, Objective C, iOS
This week we will learn three new patterns, the singleton, the facade and the Command.
Singleton: The Singleton Pattern ensures a class has only one instance, and provides a global point of access to it.
Facade: facade pattern lets you plan for changes to a sub-system.
Command: encapsulate a request in an object, allows the parameterization of clients with different requests, allows saving the requests in a queue.
Singleton pattern
Singleton pattern is a software design pattern that restricts the instantiation of a class to one object. This is useful when exactly one object is needed to coordinate actions across the system.
In Objective C:
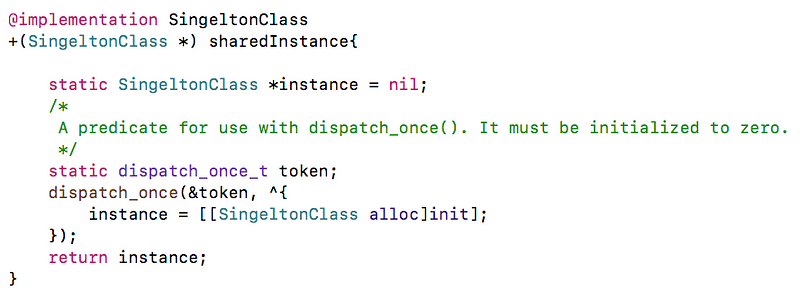
In Swift:
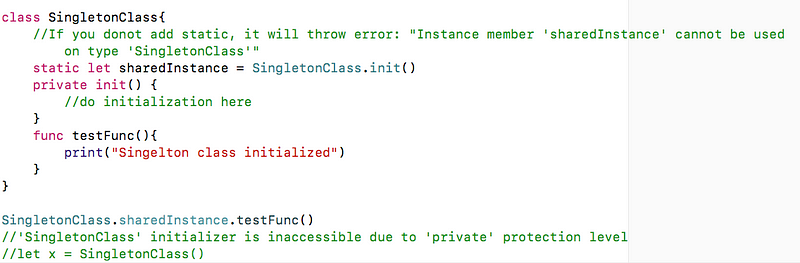
Common uses
- The abstract factory, builder, and prototype patterns can use singletons in their implementation.
- Facade objects are often singletons because only one facade object is required.
- State objects are often singletons.
- Singletons are often preferred to global variables because:
1. They do not pollute the global namespace (or, in languages with namespaces, their containing namespace) with unnecessary variables.[4]
2. They permit lazy allocation and initialization, whereas global variables in many languages will always consume resources.
Facade pattern
A facade is an object that serves as a front-facing interface masking more complex underlying or structural code.
One example of this in iOS is the UIImage class, This class is a façade which provides an interface for using and loading images that can be vector-based or bitmap-based, So no matter what type of image the application is using, it can use UIImage and have no knowledge of what’s happening underneath the class.

In Objective C:
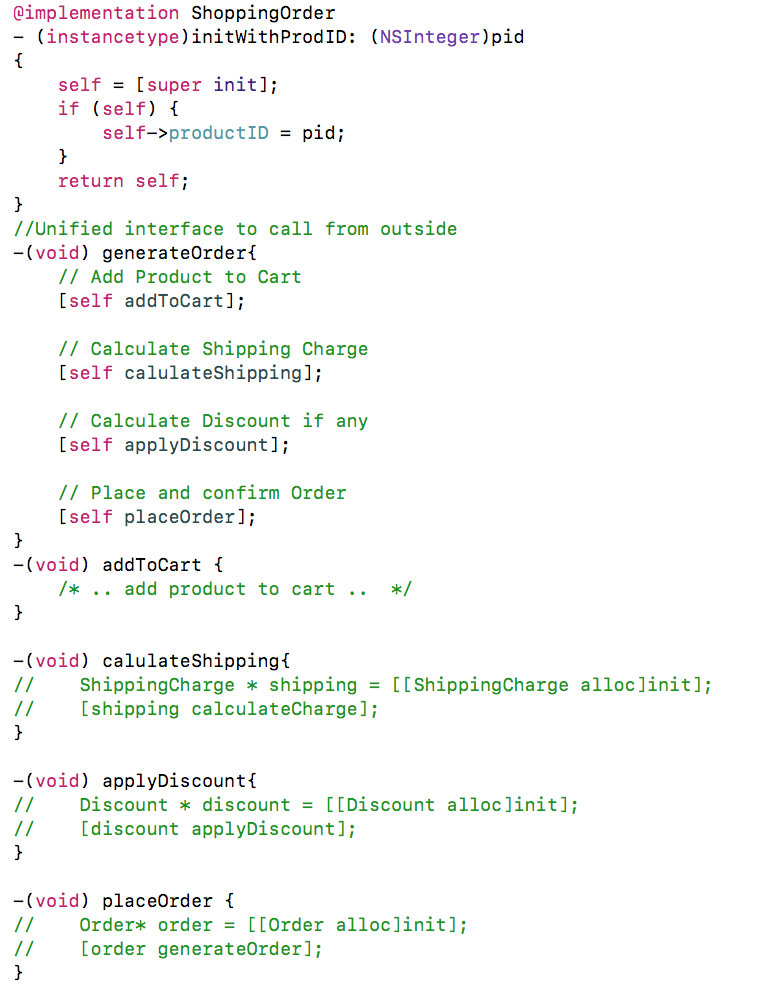
In Swift:
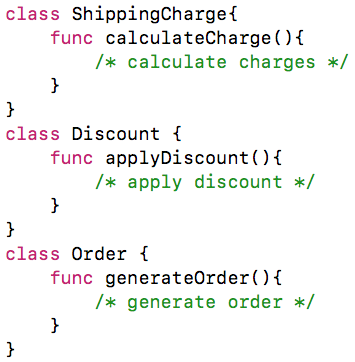

Command pattern
Command pattern is a behavioral design pattern in which an object is used to encapsulate all information needed to perform an action or trigger an event at a later time.
What problems can the Command design pattern solve?
- Coupling the invoker of a request to a particular request should be avoided. That is, hard-wired requests should be avoided.
- It should be possible to configure an object (that invokes a request) with a request.
Implementing (hard-wiring) a request directly into a class is inflexible because it couples the class to a particular request at compile-time, which makes it impossible to specify a request at run-time.
What solution does the Command design pattern describe?
- Define separate (command) objects that encapsulate a request.
- A class delegates a request to a command object instead of implementing a particular request directly.
This enables one to configure a class with a command object that is used to perform a request. The class is no longer coupled to a particular request and has no knowledge (is independent) of how the request is carried out.
In Swift: Let’s create a protocol for command and switching functionality. Assume there are two devices which support turn on/off functionality for ex: Air conditioner, Air purifier.

We have An interface that defines actions that the receiver can perform — Switchable.
As there are two types of command available for each devices, we need to implement concrete commands for each devices such as below:
The Command for turning on/off the device - ConcreteCommand
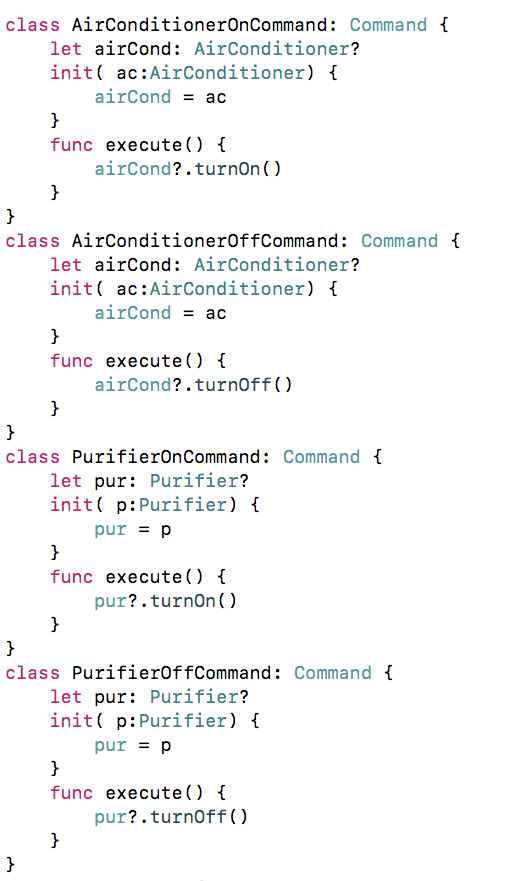
The test class or client
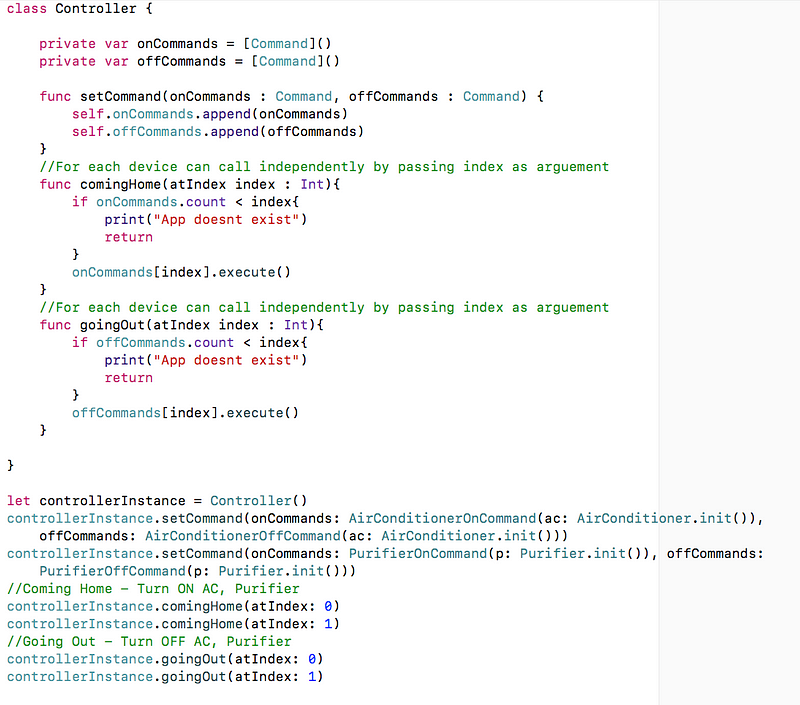
By implementing command pattern , Controller class is unaware of device-types and its implementation but provide interface for handling device.
Hope you like it, Please feel free to comment or clap to encourage :)
References: