Top IOS Interview Questions and Answers (Nov 2018) Part 1
Top IOS Interview Questions and Answers (Nov 2018) Part 1
senior iOS developer interview questions, interview questions, experienced ios interview questions
Lets start :)
Q1. How would you Persist File References in UserDefaults i.e. To store a reference to a file so that even if it move from one folder to another, you can access it?
Solution: A file URL specifies a location in the file system. If you use
the
set(_:forKey:)
method to store the location for a particular file and the user
moves that file, your app may not be able to locate that file on
next launch. To store a reference to a file by its file system
identity, you can instead create
NSURL
bookmark
data using the
bookmarkData(options:includingResourceValuesForKeys:relativeTo:)
method and persist it using the
set(_:forKey:)
method. You can then use the
URLByResolvingBookmarkData:options:relativeToURL:bookmarkDataIsStale:error:
method to resolve the bookmark data stored in user defaults to a
file URL.
Q2. What are the costs to storing things in NSUserDefaults vs something like CoreData or SQLite?
Solution: There’s basically two costs to storing things in NSUserDefaults:
1) Either all of it is in memory or none of it is (technically if you use -initWithSuiteName: you can manage that separately, but the normal case it’s all or nothing). So if you have a bunch of irrelevant stuff in your preferences, it’ll get loaded up alongside the tiny boolean you checked.
2) Similarly, it all gets updated at once. If you set that tiny boolean, the whole file is going to get saved out to disk again (though: it saves it to disk later, in a background program, so it won’t necessarily slow your program down. Still not free though).
With the tiny amount you’re storing in it, none of this should matter for you. If you end up storing more though, these two places (memory usage, and time when setting new values) are where you’ll pay the costs. Reading values from user defaults is almost always very fast, pretty much regardless of how much you store.
One other random NSUserDefaults performance tip: setting a value inside a collection inside user defaults is more expensive than setting the same value directly in user defaults. Keeping things ‘flat’ like this helps.
Q3. How exactly does
@synchronized
work?
Solution: Once you
look at the source, you’ll see that there’s a lot more going on. Apple is using
up to three lock/unlock sequences, partly because they also add
exception unwinding. This would be a slowdown compared to the much faster spinlock
approach. Since setting the property usually is quite fast,
spinlocks are perfect for the job.
@synchonized(self)
is good when you need to ensure that exception can be thrown
without the code deadlocking.
To find out what
@synchronized
does, and I saw something like this:
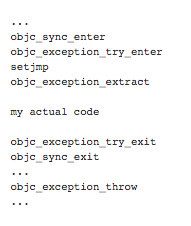
Q4. Difference b/w scheduledTimerWithTimeInterval & timerWithTimeInterval ?
Solution: Scheduled timers are automatically added to the run
loop, unscheduled timers are not.
The timer functionality
is the same.
So if a timer is not part of the default run loop (timerWithTimInterval) what triggers it?
the method addTimer:forMode: of NSRunLoop
In more descriptive way:
scheduledTimerWithTimeInterval:invocation:repeats: and scheduledTimerWithTimeInterval:target:selector:userInfo:repeats:
create timers that get automatically added to an NSRunLoop, meaning that you don’t have to add them yourself. Having them added to an NSRunLoop is what causes them to fire.
timerWithTimeInterval:invocation:repeats: and timerWithTimeInterval:target:selector:userInfo:repeats:
you have to add the timer to a run loop manually, with code like this:
[[NSRunLoop mainRunLoop] addTimer:repeatingTimer forMode:NSDefaultRunLoopMode];
Q5. Which is faster — NSArray versus NSSet ?
Solution: Yes, NSArray is faster than NSSet for simply holding and iterating. As little as 50% faster for constructing and as much as 500% faster for iterating. Lesson: if you only need to iterate contents, don’t use an NSSet.
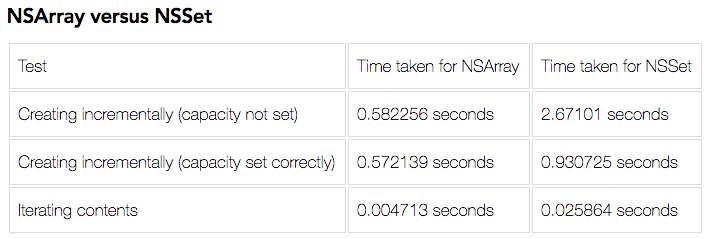
Of course, if you need to test for Lookup, work hard to avoid NSArray. Even if you need both iteration and Lookup testing, you should probably still choose an NSSet. If you need to keep your collection ordered and also test for Lookup, then you should consider keeping two collections (an NSArray and an NSSet), each containing the same objects.
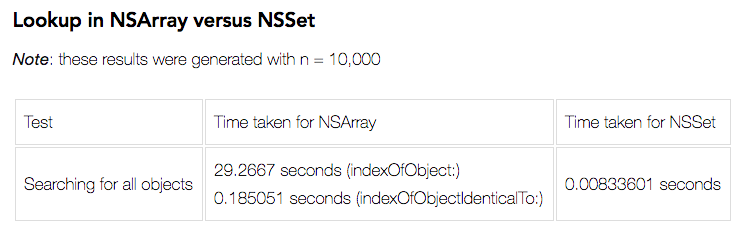
Q6. What is the entry point of swift code execution?
Solution: For iOS apps the default for new iOS project templates is to add @UIApplicationMain to a regular Swift file. This causes the compiler to synthesize a main entry point for your iOS app, and eliminates the need for a “main.swift” file.
Swift is designed to make it easy to experiment in a playground or to quickly build a script. A complete program can be a single line of code. Of course, Swift was also designed to scale to the most complex apps you can dream up. With “main.swift” you can take complete control over initialization or you can let @UIApplicationMain do the startup work for you on iOS.
References: