Operation and Grand Central Dispatch interview questions (Part II)
Operation and Grand Central Dispatch interview questions (Part II)
iOS interview question, gcd interview question, multithreading ios interview question, iOS
In this article, I am going to cover some questions which are related to Dispatch, but solutions are given in objective C language and same can be ported on Swift.
Reason behind giving solution in objective C for these questions is one of my friend given interview in Citrix, etc and they asked to write solution in objective C as opening is for developer who knows both — obj C and Swift.
Hope it helps other developers too, lets begin..
Q1. What is the Use of dispatch_once & dispatch_once_t , write down the code with example?
Solution: Singletons

Q2. What is the Use of dispatch_after , write down the code with example?
Solution: If you need something to run at a specific point in time, dispatch_after may be the right thing, though. Be sure to check out NSTimer, too.

Q3. What is Target queues , write down the code with example?
Solution:
Surprise: you’re already using them! Any queue you create must have a target queue. By default, this is set to the DISPATCH_QUEUE_PRIORITY_DEFAULT global concurrent queue.
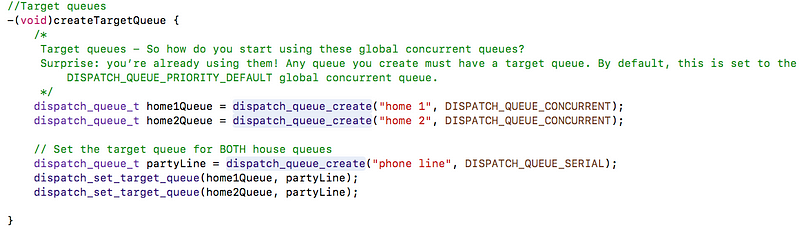
Q4. What is the problem in below code and how can you solve it?

Solution:
dispatch_set_target_queue makes every block executed from firstQueue or secondQueue behave “as if” it were submitted to concurrentQueue using dispatch_async.
Being a target queue has no effect on the semantics of dispatch_barrier_* functions. Barriers guarantee that nothing else on the queue is executing while the barrier block is running.

Q5. Write the code for creating all type of queues and let us know the priority also?
Solution:
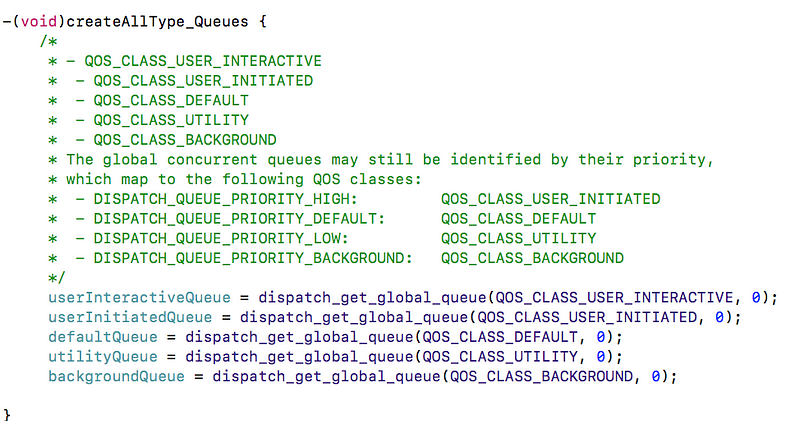
Q6. Write the code for One Resource, Multiple Readers, and a Single Writer (Basically reader writer for multi thread reading and single thread writing)?
Solution: A optimization of that sync/async pattern is the reader-writer pattern, where concurrent reads are permissible but concurrent writes are not. Thus, you’ll use a concurrent queue, dispatch_barrier_async the writes (achieving serial-like behavior for the writes), but dispatch_sync the reads (enjoying concurrent performance with respect to other read operations).

Q7. Write the code for deadlock condition ?
Solution:
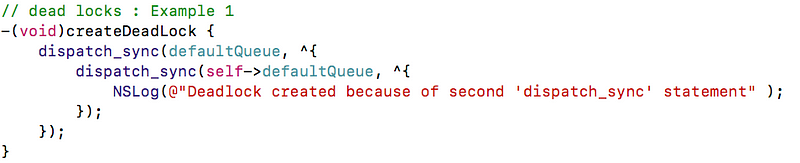
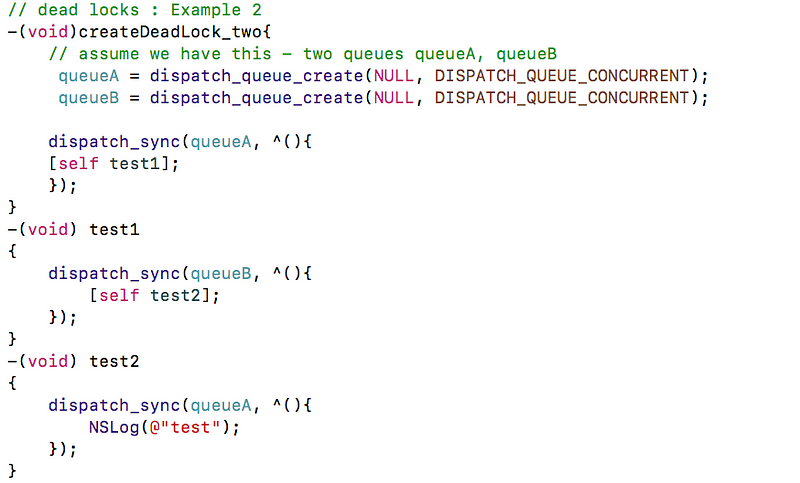
Q8. What is dispatch_apply ? Write the code for its use case?
Solution: dispatch_apply — Iterative Execution performance improvement, check below example for more clarity.

Q9. Write the code for DispatchGroup use case?
Solution:

Q10. Write the code for DispatchGroup use case?
Solution:

Q11. Write the code for DispatchSource use case?
Solution:

Q12. Write the code for DispatchData use case?
Solution:
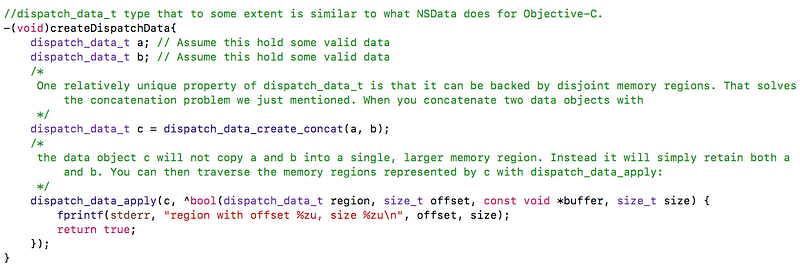