MVC vs. MVP vs. MVVM
MVC vs. MVP vs. MVVM
In this article, I am going to cover the difference between MVC, MVP and MVVM which normally asked to most of iOS engineer’s during their interviews.
In the MVC, the Controller is responsible for determining which View to display in response to any action including when the application loads.
Here, our Views have direct access to Models. Exposing the complete Model to the View, however, may have security and performance costs, depending on the complexity of our application.
MVP in iOS
In MVP, the Presenter contains the UI business logic for the View. All invocations from the View delegate directly to Presenter. The Presenter is also decoupled directly from the View and talks to it through an interface. This is to allow mocking of the View in a unit test. One common attribute of MVP is that there has to be a lot of two-way dispatching. For example, when someone clicks the “Save” button, the event handler delegates to the Presenter’s “OnSave” method. Once the save is completed, the Presenter will then call back the View through its interface so that the View can display that the save has completed.
A typical iOS application is being built around a central UIViewController class, which has many responsibilities and so is the most attractive place to put UI logic and a part of the application logic. However, we mentioned above that due to a strong cohesion between the View and the Controller (in the context of iOS UIViewController and UIView) it is convenient to think about them as a View.
Unlike MVVM, there isn’t a mechanism for binding Views to ViewModels, so we instead rely on each View implementing an interface allowing the Presenter to interact with the View.
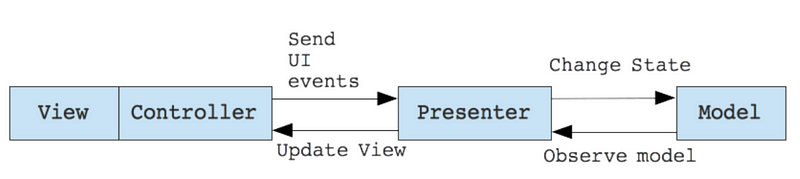
Two primary variations
Passive View: The View is as dumb as possible and contains almost zero logic. The Presenter is a middle man that talks to the View and the Model. The View and Model are completely shielded from one another. The Model may raise events, but the Presenter subscribes to them for updating the View. In Passive View there is no direct data binding, instead the View exposes setter properties which the Presenter uses to set the data. All state is managed in the Presenter and not the View.
- Pro: maximum testability surface; clean separation of the View and Model
- Con: more work (for example all the setter properties) as you are doing all the data binding yourself.
Supervising Controller: The Presenter handles user gestures. The View binds to the Model directly through data binding. In this case it’s the Presenter’s job to pass off the Model to the View so that it can bind to it. The Presenter will also contain logic for gestures like pressing a button, navigation, etc.
- Pro: by leveraging data binding the amount of code is reduced.
- Con: there’s less testable surface (because of data binding), and there’s less encapsulation in the View since it talks directly to the Model.
MVVM in iOS
In Cocoa there is its own Data Binding mechanism, but, in CocoaTouch, there isn’t. We could do only with KVO, but this thing is not convenient to use and allows you to implement only a unilateral binding. Data Binding, in its turn, makes it possible to implement the full potential inherent in MVVM and facilitate the development in general. So you should use some third-party libraries that provide Data Binding to CocoaTouch, or reactive programming. I have written generic dynamic for adding binding between ViewModel and View.
UIViewController in a MVVM and MVP is regarded as a part of View.
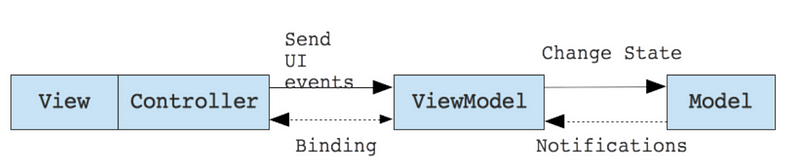
So, MVVM and MVP (Humble View) differ mostly in a Presentation layer (in MVP it is presented by a Presenter and in the MVVM by a ViewModel). The advantage of the MVVM over MVP (Humble View) is that the Presentation layer is completely independent of the View (which means much easier testing) and DataBinding usage. Together it becomes a more attractive candidate for use in modern IDE, and reduces the amount of code for synchronizing the View with the ViewModel).
The disadvantage of the MVVM is mostly in DataBinding mechanisms, as in certain situations, it may require significant memory resources, and also is a weak spot for the Memory Leak emergence.