Implementing observer pattern using Swift generics and closures
Implementing observer pattern using Swift generics and closures
Binding between ViewModel and UIView
In this post, I’ll present simple binding mechanism by implementing observer pattern using Swift generics and closures.
Objects of basic data types and values of primitive types do not provide us a way to observe their changes. In order to do that we would have to gain control of their setters (or of that which sets them) and in there notify those who are interested. Fortunately, Swift is smart enough not to allow that. Having that level of freedom would quickly go wrong. However it is possible to create our own data types and tailor them by our desires. We could make them encapsulate basic data or primitive type. That would make modifying value of encapsulated type go through our type’s interface and that’s where we can do what pleases us.
I’ve used
typealias
command to introduce new type,
Observer, which is a closure that accepts one argument and returns
nothing. With that type we declared a
observer
property, making it an Optional so it’s not required to be set.

Next, we’ve created a setter for observer property just to make syntax a bit nicer. Finally, we’ve changed property observer to call that observer closure if it is set. That’s it! Let’s go through the example.
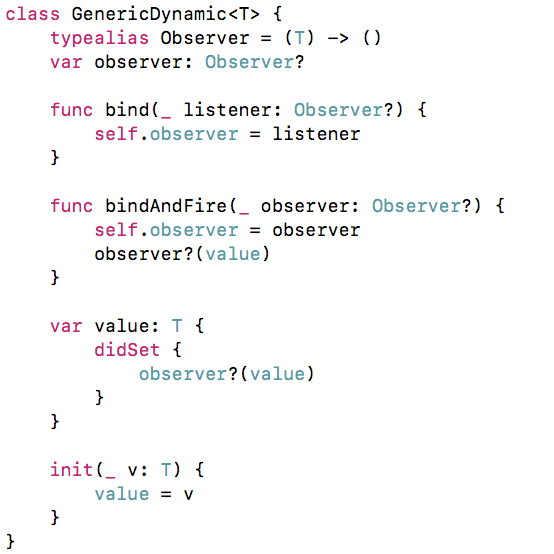
Now we are ready with basic template of generic dynamic class which can be used to define custom type for binding purpose between viewModel and Views.
Lets try to understand the use case for it, Consider a viewModel class which has bool variable named status which can be true or false and based on its state, a button in Xib need to enable and disable, There are several ways to do this thing, in this article we are covering how to create binding between status variable and button UI element.

In the above image , default initialization of status variable has been shown and during run time we have changed the status var like below:

still we have not bind the button with status, so lets see how we will do it.

Now when ever there is a change in status var of viewModel class, our button enable state will update accordingly.
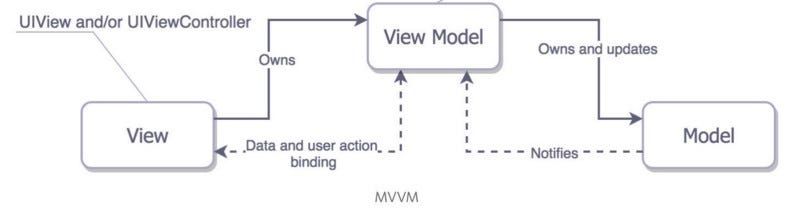
Hope this clarify the use case of observer pattern, This above example shows use-case in MVVM based app architecture.