Top IOS Interview Questions and Answers (August 2018) Part 2
Top IOS Interview Questions and Answers (August 2018) Part 2
Q1. Why @property/@synthesize does not exist in swift?
Swift provides no differentiation between properties and instance variables (i.e, the underlying store for a property). To define a property, you simply declare a variable in the context of a class.
If you have experience with Objective-C, you may know that it provides two ways to store values and references as part of a class instance. In addition to properties, you can use instance variables as a backing store for the values stored in a property.
Swift unifies these concepts into a single property declaration. A Swift property does not have a corresponding instance variable, and the backing store for a property is not accessed directly. This approach avoids confusion about how the value is accessed in different contexts and simplifies the property’s declaration into a single, definitive statement. All information about the property — including its name, type, and memory management characteristics — is defined in a single location as part of the type’s definition.
Q2. How optionals are implemented?
Optionals are implemented as
enum
type in
Swift.
See Apple’s Swift Tour for an example of how this is done:
enum OptionalValue<T> {
case None
case Some(T)
}
Q3. Declare some constant variables and initialize during instance creation of that class?
For class instances, a constant property can be modified during initialization only by the class that introduces it. It cannot be modified by a subclass.
You can assign a value to a constant property at any point during initialization, as long as it is set to a definite value by the time initialization finishes. Once a constant property is assigned a value, it can’t be further modified.
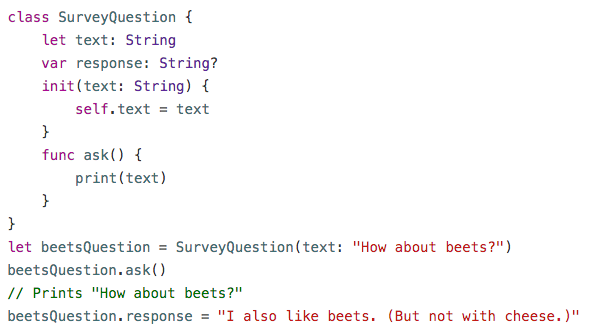
Q4. Define a variable and when ever you set that variable , its retain count increase by 4, How will you do it?
var vc1:NSDate? = NSDate()
print(CFGetRetainCount(vc1)) // 2 - I expected this to be 1 as only one variable is strongly referring this object.
var vc2:NSDate? = vc1
print(CFGetRetainCount(vc1)) // 3 - reference count incremented by 1 (strong reference)
var vc3:NSDate? = vc2
print(CFGetRetainCount(vc3)) // 4 - reference count incremented by 1 (strong reference)
Q5. Why outlets are weak?
The most common one, the one Apple uses in its sample code, follows this pattern:
@IBOutlet private weak var someLabel: UILabel!
@IBOutlet private weak var someLabel: UILabel?
@IBOutlet private var someLabel: UILabel?
These examples all follow 3 simple rules:
-
!
needs a guarantee that the view exists, so always usestrong
to provide that guarantee -
If it’s possible the view isn’t part of the view hierarchy,
use
?
and appropriate optional-handling (optional binding/chaining) for safety -
If you don’t need a view anymore after removing it from the
view hierarchy, use
weak
so it gets removed from memory.
The type qualifier
IBOutlet
is a
tag applied to an property declaration so that the Interface
Builder application can recognize the property as an outlet and
synchronize the display and connection of it with Xcode.
Q6. How will you modify let variables in init function?
class Point {
let x: Int
let y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
let p1 = Point(x: 1, y: 1)
Q7. How do you define Implicitly unwrapped optionals ?
When we define an Implicitly unwrapped optional, we define a container that will automatically perform a force unwrap each time we read it.
var text: String! = "Hello"
If now we read
text
let name = text
we don’t get an Optional
String
but a
plain
String
because
text
automatically unwrapped it's content.
However text is still an optional so we can put a
nil
value
inside it
text = nil
But as soon as we read it (and it contains
nil
) we get a
fatal error because we are unwrapping an optional containing
nil
let anotherName = text
fatal error: unexpectedly found nil while unwrapping an Optional value
Hope you like this article & is useful for people looking to find out the recently asked iOS interview questions, Please ❤️ to recommend this post to others 😊. Let me know your feedback. :)