Top IOS Interview Questions and Answers (August 2018)
Top IOS Interview Questions and Answers (August 2018)
August 2018 iOS latest interview questions
In this article, I am going to cover some of the questions asked to iOS engineer in recents interview(August 2018). Those who are preparing , it will surely help them.
Q1. What is the difference between swift and objective C?
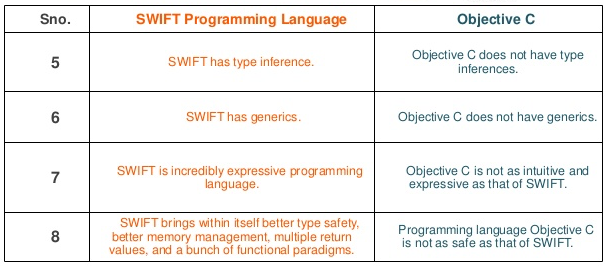
Q2. What is manual retain cycle?

Q3. How does memory management work in Swift?
We start by looking at the basics of memory management in Swift. ARC (automatic reference counting) does most of the memory management work for you, which is very good news. The principle is very simple: By default, each reference, that points to an instance of a class, is a so-called strong reference. As long as there is at least one strong reference pointing to an instance, this instance will not be deallocated. When there’s no strong reference pointing to that instance left, the instance will be deallocated. Let’s take a look at the following example:
class TestClass {
init() {
print(“init”)
}
deinit {
print(“deinit”)
}
}
var testClass: TestClass? = TestClass()
testClass = nil
After creating the instance, the situation looks as follows:

testClass has a strong reference to an instance of TestClass. If we now set this reference to nil, the strong reference is gone and since there is no strong reference left, the instance of TestClass gets deallocated:
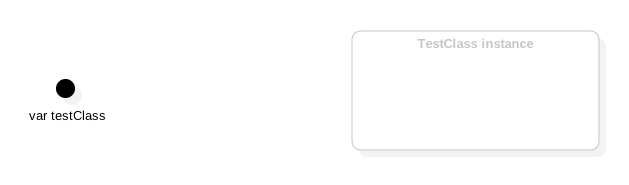
By the way, if you take a look at the console, you can see that everything is working fine because the deinit method will only be called by the system when the instance gets deallocated:

If the instance of TestClass was not deallocated, there wouldn’t be the message “deinit”. As we will discuss later, placing a log message inside of deinit is a very good way to observe the deallocation of an object.
Q4. What methods are used to claim ownership(Increase retain count by 1)?
alloc/init — Create an object and claim ownership of it
retain — Used to claim ownership of that object
copy — Copy an object and claim ownership of it
Q5. What methods are used to declaim ownership(Decrease retain count by 1)?
release — Declaim ownership of an object.
autorelease — Declaim ownership of an object.
Q6. What is protocol oriented programming and what its benefit?
At the heart of Swift’s design are two incredibly powerful ideas: protocol-oriented programming and first class value semantics. Each of these concepts benefit predictability, performance, and productivity, but together they can change the way we think about programming. Find out how you can apply these ideas to improve the code you write.
protocol oriented programming in swift
Q7. How will you check optional method of a protocol is implemented in inherited class?
You can use the
is
and
as
operators
described in Type Casting
to check for protocol conformance, and to cast to a specific
protocol. Checking for and casting to a protocol follows exactly
the same syntax as checking for and casting to a type:
-
The
is
operator returnstrue
if an instance conforms to a protocol and returnsfalse
if it does not. -
The
as?
version of the downcast operator returns an optional value of the protocol’s type, and this value isnil
if the instance does not conform to that protocol. -
The
as!
version of the downcast operator forces the downcast to the protocol type and triggers a runtime error if the downcast does not succeed.
Q9. Structure has default parameterized init function, I want to have both parameterized and empty constructor, how is it possible?

Q10. What is the difference in struct and classes and where will you use structure?
Classes have additional capabilities that structures don’t have:
- Inheritance enables one class to inherit the characteristics of another.
- Type casting enables you to check and interpret the type of a class instance at runtime.
- Deinitializers enable an instance of a class to free up any resources it has assigned.
- Reference counting allows more than one reference to a class instance.

Q11. I have a structure of 1000 var in it, I want to pass structure from one class to another class, as it’s value type, how will you do it?
Structures and Enumerations Are Value Types
A value type is a type whose value is copied when it’s assigned to a variable or constant, or when it’s passed to a function BUT
Collections defined by the standard library like arrays, dictionaries, and strings use an optimization to reduce the performance cost of copying. Instead of making a copy immediately, these collections share the memory where the elements are stored between the original instance and any copies. If one of the copies of the collection is modified, the elements are copied just before the modification. The behavior you see in your code is always as if a copy took place immediately.
Q12. What is MVVM and how it’s different from MVC?
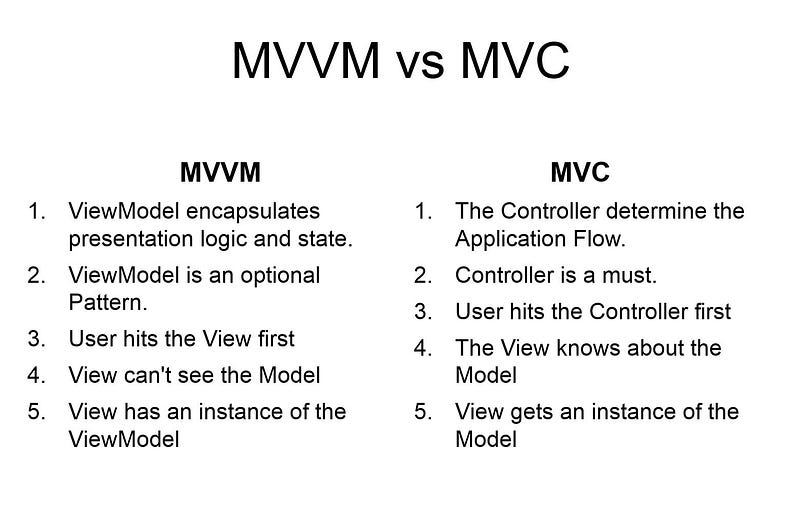
Q13. Write a code for creating strong reference cycle?
The strong reference cycle prevents the
Person
and
Apartment
instances from ever being deallocated, causing a memory leak in
your app.
Example 1:

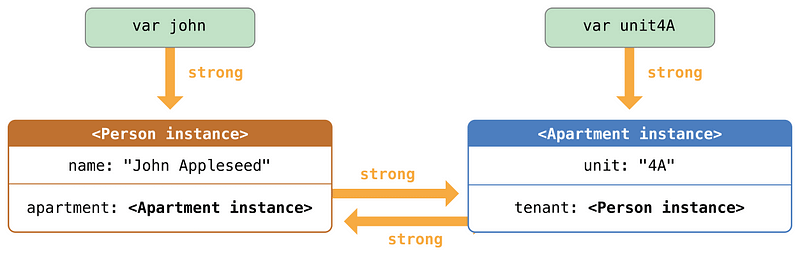
Example 2:
The example below shows how you can create a strong reference
cycle when using a closure that references
self
. This
example defines a class called
HTMLElement
,
which provides a simple model for an individual element within
an HTML document:
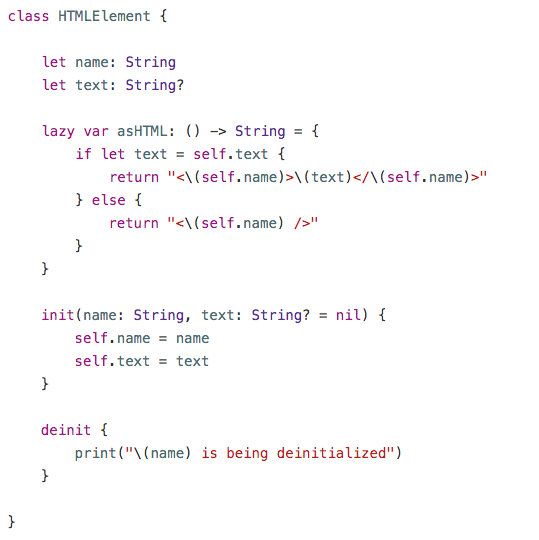
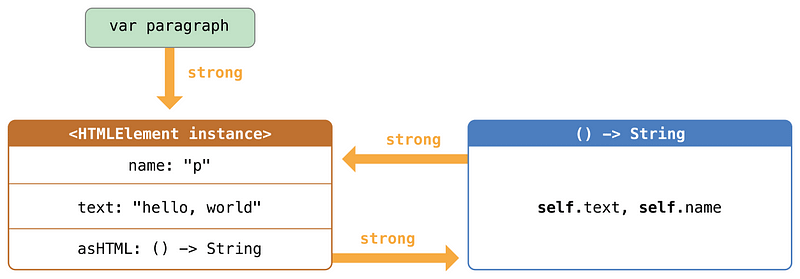
Q14. Using operation and Operation queue — how will you run 5 parallel thread and once all thread completes..How will you notify that all tasks completed?
Sol1: create extra operation and have all other operation as dependency to it, once all 5 completed, it will be executed.
Sol2: have operation count, once its reduced to 0, perform complete operation.
Q15. What is core data stack and how parent child context works?
Q16. What is the difference between NSArray and Array?
Array
is a
struct,
therefore it is a
value type
in Swift.
NSArray
is an
immutable Objective C
class,
therefore it is a
reference type
in Swift and it is bridged to
Array<AnyObject>
.
NSMutableArray
is the mutable subclass of
NSArray
.
Q17. How collections are implemented in swift?
Swift provides three primary collection types, known as arrays, sets, and dictionaries, for storing collections of values. Arrays are ordered collections of values. Sets are unordered collections of unique values. Dictionaries are unordered collections of key-value associations.
Swift’s array, set, and dictionary types are implemented as generic collections. For more about generic types and collections
NOTE
-
Swift’s
Array
type is bridged to Foundation’sNSArray
class.
2. Swift’s
Set
type is
bridged to Foundation’s
NSSet
class.
3. Swift’s
Dictionary
type
is bridged to Foundation’s
NSDictionary
class.
Q18. How will you store custom objects in userdefault?
If you have tried to save custom object into UserDefaults
before, you might get an error like this:'NSInvalidArgumentException', reason: 'Attempt to
insert non-property list object
According to Apple’s documentation on UserDefaults,
A default object must be a property list — that is, an instance of (or for collections, a combination of instances of) NSData, NSString, NSNumber, NSDate, NSArray, or NSDictionary.
Many of you will be starting your journey of Swift data
serialization with some incredibly powerful and easy-to-use
tools. I, of course, am referring to the
Codable
protocol which was introduced in Swift 4 to save developers from
the agony that is de/serializing
Data
.
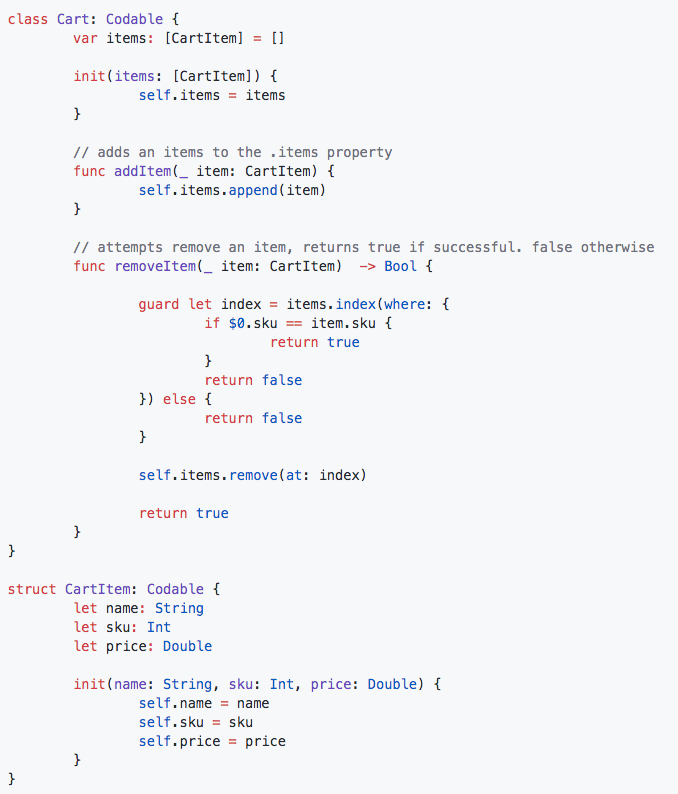
Q19. What is the use of plist?
In the context of iPhone development, Property Lists are a key-value store that your application can use to save and retrieve persistent data.
Q20. Closure and its closure types with examples
Read this for more detail Closures
- Simple closure
- Closure that contains statements
- Closure that accepts parameter
- Closure that returns value
- Passing closures as a function parameter
- Trailing closure
- Autoclosure
- Auto-closure with arguments and return value
- Escaping vs No escaping closures
- No escaping closure
- Escaping closure
Hope you like this article & is useful for people looking to find out the recently asked iOS interview questions, Please ❤️ to recommend this post to others 😊. Let me know your feedback. :)