iOS interview questions(9)
iOS 2018 Series: Cracking iOS interview or Become iOS expert (9)
Fast Enumerations, Toll-Free Bridging & Must know iOS Basics
This time I am going to cover some must know things about iOS and in general and covering generics, LRU Cache in next post.
Fast Enumeration
Fast enumeration is the preferred method of enumerating the contents of a collection because it provides the following benefits:
-
The enumeration is more efficient than using
NSEnumerator
directly. - The syntax is concise.
- The enumerator raises an exception if you modify the collection while enumerating.
- You can perform multiple enumerations concurrently.
Toll-Free Bridging
There are a number of data types in the Core Foundation framework and the Foundation framework that can be used interchangeably. This capability, called toll-free bridging, means that you can use the same data type as the parameter to a Core Foundation function call or as the receiver of an Objective-C message. ex: NSArray and CFArrayRef can be used interchangeably between Core Foundation and Foundation
Core Foundation type CFArrayRef
Foundation class NSArray
Explain the proper uses of (__bridge), (__bridge_retained) and (__bridge_transfer) casts.
(__bridge T) op
casts the operand to the destination type T. If T is a
retainable object pointer type, then op must have a
non-retainable pointer type. If T is a non-retainable pointer
type, then op must have a retainable object pointer type.
Otherwise the cast is ill-formed. There is no transfer of
ownership, and ARC inserts no retain operations.
(__bridge_retained T) op
casts the operand, which must have retainable object pointer
type, to the destination type, which must be a non-retainable
pointer type. ARC retains the value, subject to the usual
optimizations on local values, and the recipient is responsible
for balancing that +1.
(__bridge_transfer T) op
casts the operand, which must have non-retainable pointer type,
to the destination type, which must be a retainable object
pointer type. ARC will release the value at the end of the
enclosing full-expression, subject to the usual optimizations on
local values.
Why Does the App crashes on Low Device Memory?
iOS Devices Use virtual memory with Paging . As it is a Mobile device and there is no Extensible memory or pretty large Memory available (Like Hard drives) , so the availability of the pages is limited by various factors such as the number of applications open , Allocations by different applications , etc. Moreover , some on-board applications will always keep using some pages even when they are in dormant state such as safari , i-tunes , messaging etc. So , essentially with number of application active , the number of pages your application can use gets diminished further.
So , your application will crash in cases when the rate of allocation by your application is exceeding the rate at which pages are being freed by other Applications.
OS only frees up read-only data from the memory while writable data is not freed-up .When the writable data crosses a certain threshold , the OS asks the application to free memory. Unable to free the memory leads to the crash.
Explain the relationship between a UIView and a CALayer
Despite what many developers think, it’s the CALayer that’s the fundamental drawing unit in iOS. The reason why we perceive UIView as such is because it’s a thin layer on top of CALayer and for most UI challenges using some form of UIView works just fine. You can create custom views, draw into them, handle user interactions, and even animate them without ever having to touch CALayer.
Every UIView comes packaged with a CALayer knows as the “backing layer” or “underlying layer.” Many of the methods you call on UIView simply delegate to the layer. When you change a view’s frame, it’s simply changing the layer’s frame. If you change the alpha, it changes the layer’s alpha…and so on with background colors, transformations and more. And while you can maintain a hierarchy of UIViews each representing parents and children of one another, you can do the same with CALayer.
Should I use subviews or sublayers?
This isn’t a simple question, but there’s one rule of thumb that make deciding a lot easier. The main different between a view and a layer is that views can accept user input while a layer cannot. A layer is simply a graphical representation. Views can handle user taps, drags, pinches, etc. To me, this is all you need to know.
conformsToProtocol vs respondsToSelector
conformsToProtocol
method let you check is your object implement specific protocol.
It doesn't tell you has it contains the method this protocol
defines (some of the method can be optional).
respondsToSelector
check is your object has a specific method (the method
doesn't need to be defined in any protocol) it can be method
defined in class.
Responder Chain and First Responder
A ResponderChain is a hierarchy of objects that have the opportunity to respond to events received.
The first object in the ResponderChain is called the FirstResponder.
Whats the difference between frame and bounds?
The bounds of an UIView is the rectangle, expressed as a location (x,y) and size (width,height) relative to its own coordinate system (0,0).
The frame of an UIView is the rectangle, expressed as a location (x,y) and size (width,height) relative to the superview it is contained within.
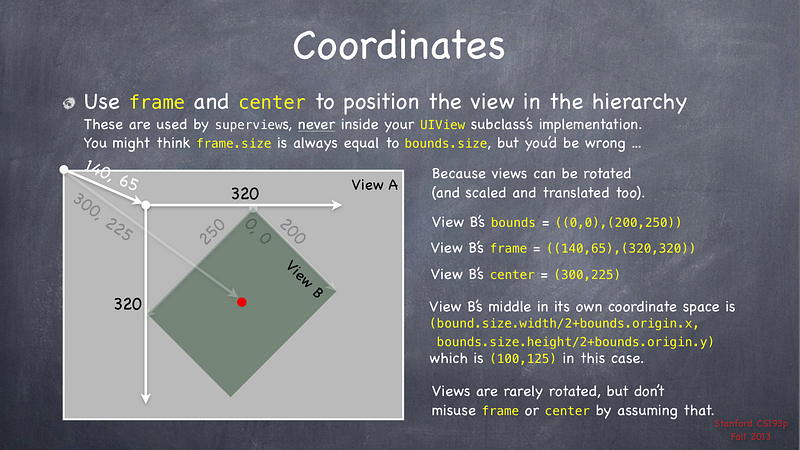
Shallow copying vs deep copy.
Copies of objects can be shallow or deep. Both shallow- and deep-copy approaches directly duplicate scalar properties but differ on how they handle pointer references, particularly references to objects (for example, NSString *str). A deep copy duplicates the objects referenced while a shallow copy duplicates only the references to those objects. So if object A is shallow-copied to object B, object B refers to the same instance variable (or property) that object A refers to. Deep-copying objects is preferred to shallow-copying, especially with value objects.
Shallow is “by Reference”, Deep is “by Value”
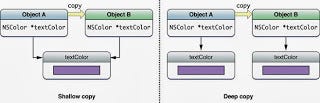
__unsafe_unretained is just like __weak but the pointer is not set to nil when the object is deallocated. Instead the pointer is left dangling.
Hope you like this article. Please ❤️ to recommend this post to others 😊. Let me know your feedback. :)