iOS interview questions (5)
iOS 2018 Series: Cracking iOS interview or Become iOS expert (5)
Chapter 5: Enums, Struct and Class
As we all know, Three big selling points of Swift are its safety, speed and simplicity.
Safety implies that it’s difficult to accidentally write code that runs amok, corrupting memory and producing hard-to-find bugs. Swift makes your work safer because it tries to make it obvious when you have a bug by showing you problems at compile time, rather than hanging you out to dry at runtime.
Moreover, because Swift lets you clearly express your intent, the optimizer can go to town to help your code run lightning fast.
The Swift language core is simple and highly-regularized, thanks to being built upon a surprisingly small number of concepts. Despite its relatively simple rules, you can do amazing things.
The key to making this happen is the Swift type system:
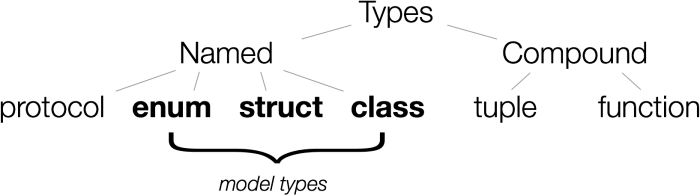
Structures and Enumerations Are Value Types
You’ve actually been using value types extensively throughout the previous chapters. In fact, all of the basic types in Swift — integers, floating-point numbers, Booleans, strings, arrays and dictionaries — are value types, and are implemented as structures behind the scenes.
Enums
Enums in Swift have a few more features like you can add an
initializer method or custom methods to extend the
functionality. If you didn’t want to select a specific member
value when creating an instance then you can provide an
init
method
which defaults to one of the member values.
enum Type {
case Friend
case Family
case Coworker
case Other
init() {
self = .Friend
}
}
Explain the difference between raw and associated values in Swift.
This question tests the developer’s understanding of enumeration in Swift. Enumeration provides a type-safe method of working with a group of related values. Raw values are compile time-set values directly assigned to every case within an enumeration, as in the example detailed below:
enum Alphabet: Int {
case A = 1
case B
case C
}
In the above example code, case “A” was explicitly assigned a raw value integer of 1, while cases “B” and “C” were implicitly assigned raw value integers of 2 and 3, respectively. Associated values allow you to store values of other types alongside case values, as demonstrated below:
enum Alphabet: Int {
case A(Int)
case B
case C(String)
}
Structures
struct Resolution {
var width = 0
var height = 0
}
All structures have an automatically-generated memberwise initializer, which you can use to initialize the member properties of new structure instances. Unlike structures, class instances do not receive a default memberwise initializer.
let vga = Resolution(width: 640, height: 480)
Identity Operators
It can sometimes be useful to find out if two constants or variables refer to exactly the same instance of a class. To enable this, Swift provides two identity operators:
-
Identical to (
===
) -
Not identical to (
!==
)
Classes
Classes and structures have a similar definition syntax. You
introduce classes with the
class
keyword
and structures with the
struct
keyword.
Both place their entire definition within a pair of braces
class VideoMode {
var resolution = Resolution()
var
interlaced = false
var frameRate = 0.0
var name:
String?
}
Choosing Between Classes and Structures
Classes have additional capabilities that structures do not:
- Inheritance enables one class to inherit the characteristics of another.
- Type casting enables you to check and interpret the type of a class instance at runtime.
- Deinitializers enable an instance of a class to free up any resources it has assigned.
- Reference counting allows more than one reference to a class instance.
Consider creating a structure when one or more of these conditions apply:
- The structure’s primary purpose is to encapsulate a few relatively simple data values.
- It is reasonable to expect that the encapsulated values will be copied rather than referenced when you assign or pass around an instance of that structure.
- Any properties stored by the structure are themselves value types, which would also be expected to be copied rather than referenced.
- The structure does not need to inherit properties or behavior from another existing type.
Bonus : SubClass vs Categories vs Extensions
Subclassing in simple words is changing the behavior of properties or methods of an existing class.
- Source code
Categories provide a way to add methods to a class even if its source code is not available to you. ex: NSString
Extensions — This includes the ability to extend types for which you do not have access to the original source code (known as retroactive modeling).
2. Instance variable/Properties
Category : Not possible, Note that in a category you can’t add an instance variable, since methods within a category are added to a class at runtime.
Extensions : Possible, Extensions can add instance variables.
3. Accessibility to Inherited classes.
Category — All methods defined inside a category are also available to the inherited class
Extensions — All properties and methods defined inside a class extension are not even available to inherited class.
Conclusion:
- Subclassing is better option if you want to customize an existing stuffs or functionalities, and Category is a best option if you want to add additional functionalities to an existing class
- Usually people will use extensions to hide private information of a class without exposing them to access from any other class.
Hope you are loving it!