Microsoft Interview Experience — Part 1 (Solution in Swift)
Microsoft Interview Experience — Part 1 (Solution in Swift)
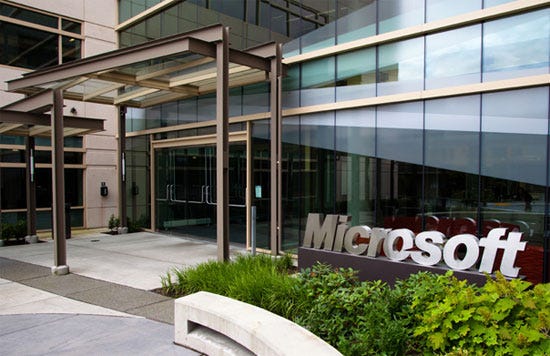
1. Given a m x n matrix, if an element is 0, set its entire row and column to 0. Do it in-place.
Example 1:
Input: [[1,0,3]]
Expected: [[0,0,0]]
Example 2:
Input: [[0,1,2,0],[3,4,5,2],[1,3,1,5]]
Expected: [[0,0,0,0],[0,4,5,0],[0,3,1,0]]
Solution:
2. Given an array of size n, find the majority element. The
majority element is the element that appears more than
⌊ n/2 ⌋
times.
You may assume that the array is non-empty and the majority element always exist in the array.
Example 1:
Input: [3,2,3]
Output: 3
Example 2:
Input: [2,2,1,1,1,2,2]
Output: 2
Solution:
3. Given sorted array of numbers and a sum. we have to find any two numbers whose sum is equal to the given sum. Time Complexity: O(n)
Solution: