Design a Chess Game
Design a Chess Game
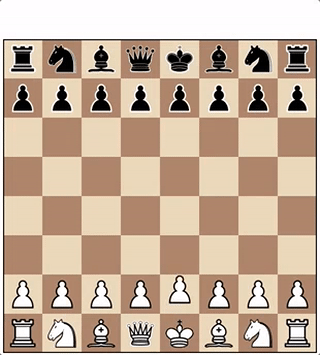
The Problem
Our goal is to implement chess classes/functions and its flow. We may/may not implement an AI for the program. Rather, this game is meant for two human opponents to play against each other but its extendible for computer as a opponent. A game of chess involves chess pieces, and a chess board.
From the application developer perspective, we should be telling the classes and its relation, class diagrams & flow. We all know the time scarcity during a technical discussion and some time its difficult what other guy is expecting from us.
Use case diagram
All systems interact with human or automated actors that use the system for some purpose, and both human and actors expect the system to behave in predictable ways.
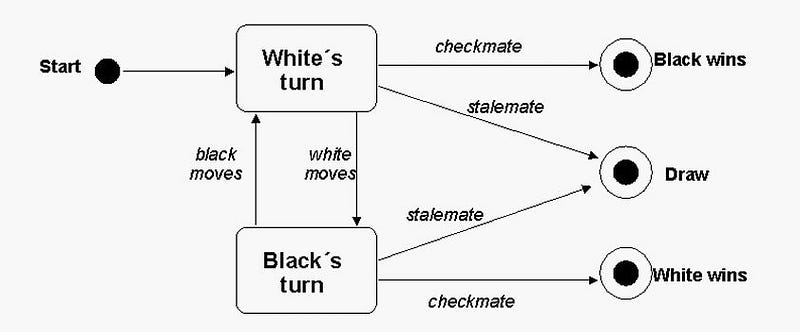
Implementation
We are going to design classes and basic functionality which can be wrapped up in library or framework and can be used by application.
Let’s start with identifying the classes involved in designing a chess game.
Directly exposed classes to application:
Game : This class is the main interface class for application to consume and utilise the business logic behind it. At the time of instantiation we need to pass few information which will play help in playing chess like firstPlayer: Player, secondPlayer: Player, board: Board = Board(state: .newGame), colorToMove: Color = .white
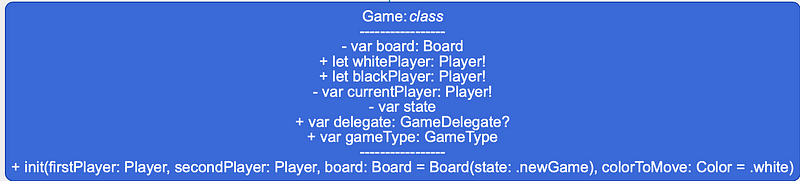
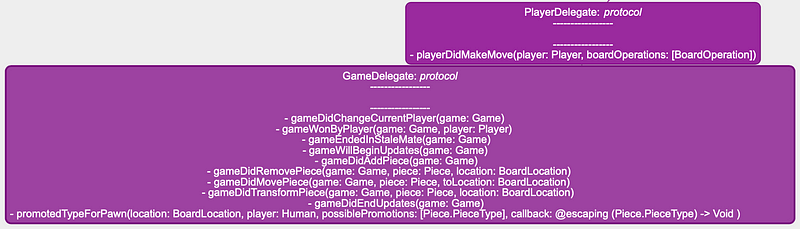
Piece : This class will be used to design piece view at the application side, based on piece.type, piece.color we can decide which icon image we need to use. It will contain a property called as movement: PieceMovement which will help in making sure the piece can move to the location based on the piece type .
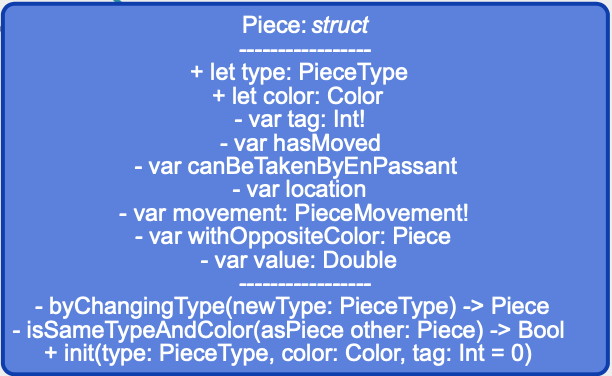
BoardLocation : This class will help in mapping the piece view with board based on location instance. To Add initial piece views, it will have all: [BoardLocation] parameter which will have 64 elements and using the index from 0..<64 range, we will map x co-ordinate as index % 8 & y co-ordinate as index / 8 , index: Int parameter.
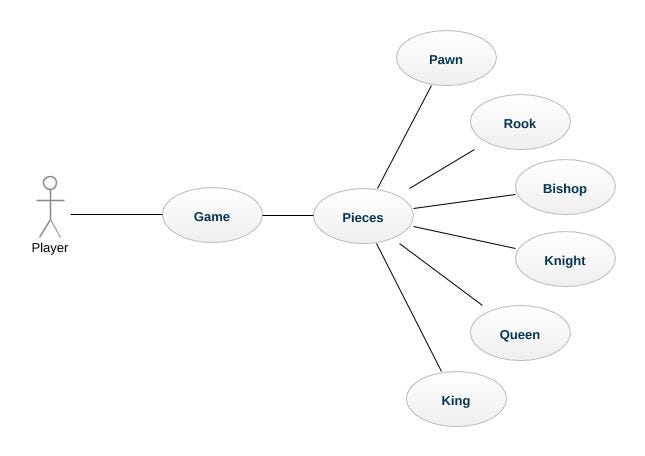
Application classes which will consume framework/library classes:
BoardView: This class will be of type View(like UIView in iOS), which will have draw(_ rect: CGRect) method for drawing 64 rectangles with two different color in a board. It will expose one callback method
func touchedSquareAtIndex(boardView: BoardView, index: Int) which will be consumed by main controller class.
PieceView: This class will be of type View, which will take piece: Piece, location: BoardLocation as a input and use piece.type, piece.color to draw images like WhiteKnight or BlackKnight.
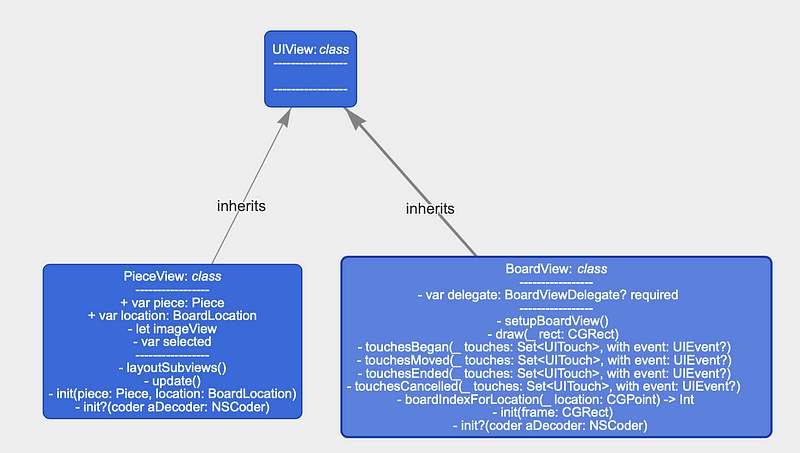
GameController: This class is responsible for drawing UI and handling its interaction, call back from board view. Call back handling detail :- If piece view is selected then it will have selectedIndex variable to update the selection UI for a peice and when empty board has been clicked to make a move , we will recieve touchedSquareAtIndex call back, will utilise the index argument to get the current board location and validates If it has tapped the same piece again, deselect it or Select new piece if possible game.currentPlayer.occupiesSquare(at: location) or If there is a selected piece, see if it can move to the new location game.currentPlayer.movePiece(from: BoardLocation(index: selectedIndex),to: location)
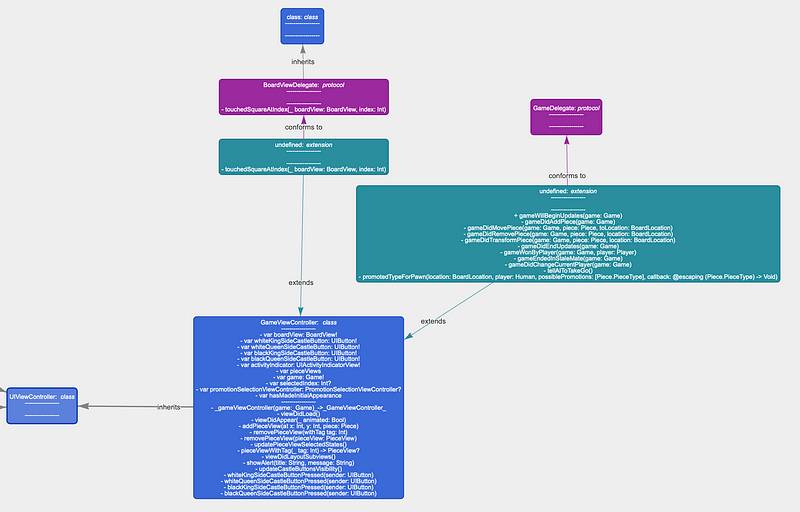
It time to move into more details of library or framework which will contain chess game classes and its functionality in detail. we have covered Game, Piece & BoardLocation already and lets explore more classes in play.
Classes:
- Board: This class will have squares array of type Square class, method for setup for NewGame like setupForNewGame and expose methods for manipulating pieces, get specific pieces & for checking check mate state.
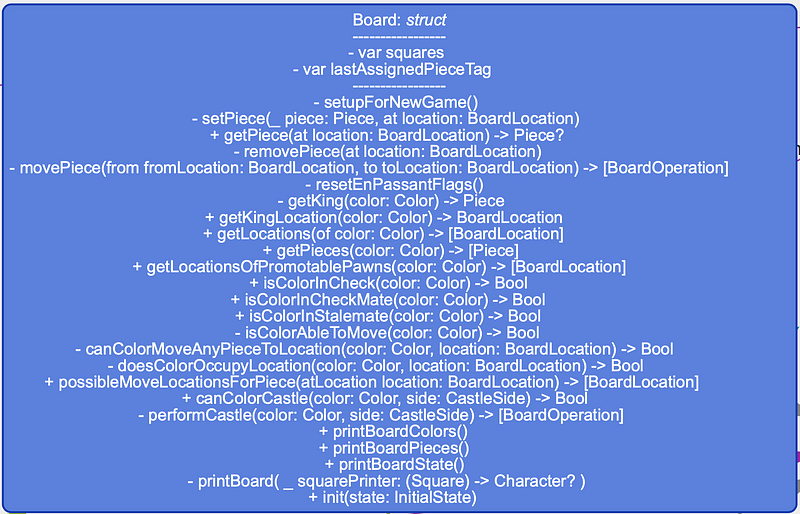
2. Square: This class hold piece: Piece object or empty.
3. PieceMovement: This is Base Class, which will work as factory and provide interface for getting pieceMovement(for pieceType: Piece.PieceType) of different type for example: PieceMovementPawn, PieceMovementKnight, PieceMovementQueen etc. It will implement few methods like canPieceMove, isMovementPossible, canPieceOccupySquare etc.
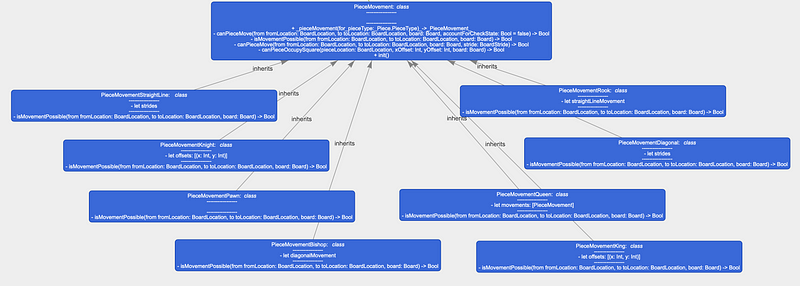
4. Player: This is base class and can be conformed by Human, AI or Computer class, it will give one call back method to inform that player made the move & expose one method moving piece movePiece/canMovePiece.
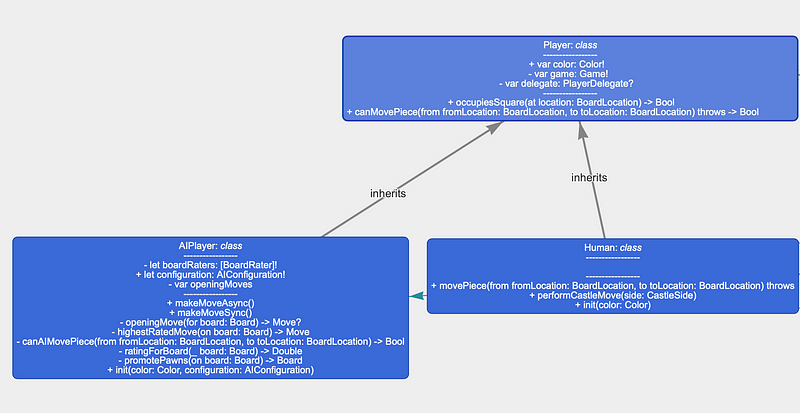
5. BoardOperation: This class will take three input type: OperationType, piece: Piece, location: BoardLocation & OperationType like movePiece, removePiece, transformPiece.
Hope you like the explanation. For more design questions , Please wait and follow me.
References: